draw parabola unity 3d c
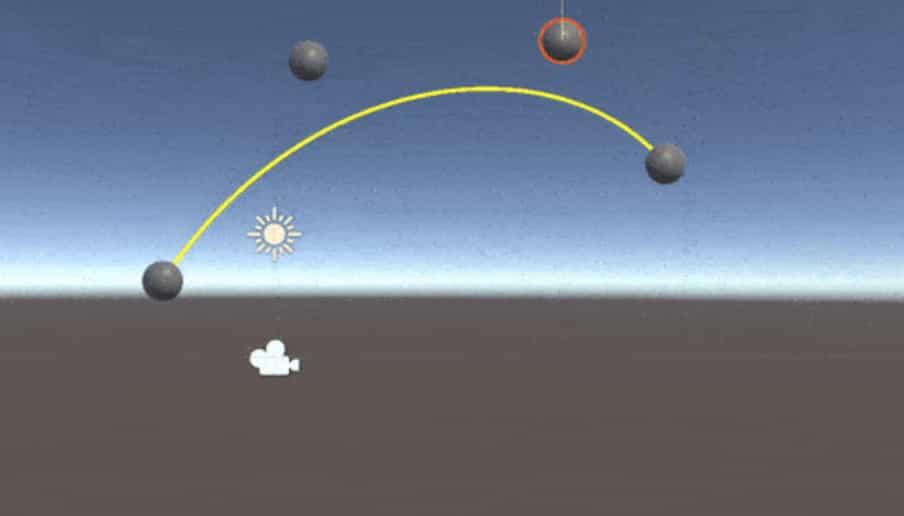
Sometimes, you need to draw lines, circles or curves in your Unity games. In these cases, y'all can utilize Unity'southward LineRenderer form. In this tutorial, we will come across how we can depict lines, polygons, circles, wave functions, Bézier Curves. And besides nosotros will see how we tin can practice a free drawing using Line Renderer in Unity3D. In society to run across our other Unity tutorials, click here.
Line Renderer Component
To describe a line we accept to add a LineRenderer component to a game object. Even if this component can be fastened to whatsoever game object, I advise yous create an empty game object and add the LineRenderer to this object.
Nosotros need a material which will be assigned to LineRenderer. To exercise this create a material for the line in Projection Tab. Unlit/Color shader is suitable for this material.
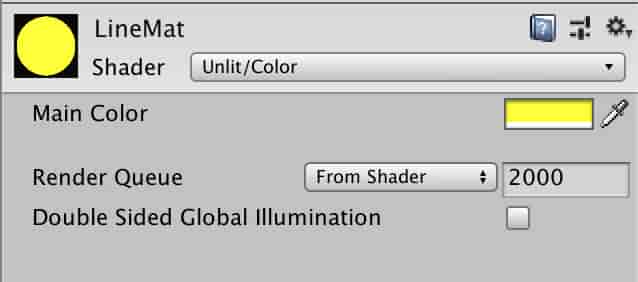
Assign LineMat textile to the Line Renderer component.
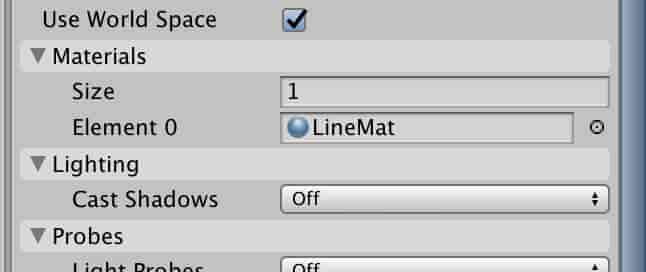
Line Renderer draws lines betwixt determined positions. In other words, we tell the Line Renderer the points which will be connected and Line Renderer connects these points.
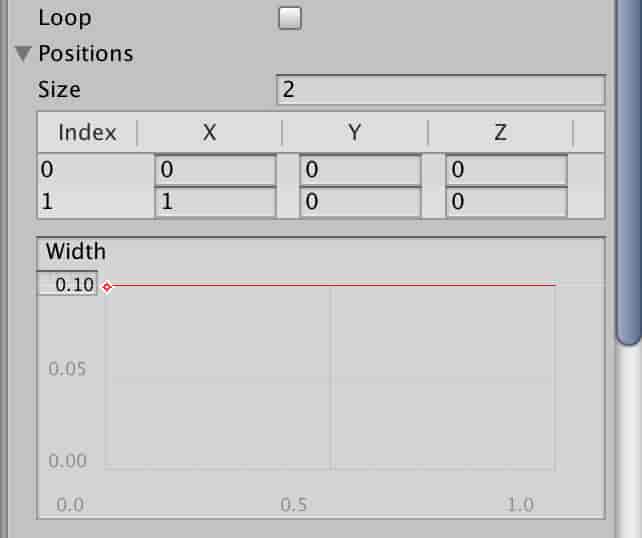
In the Positions department, you can change the number of points and positions of points. If you lot enter ii different points, you will get a directly line. You can besides change the width of the line in the section below.
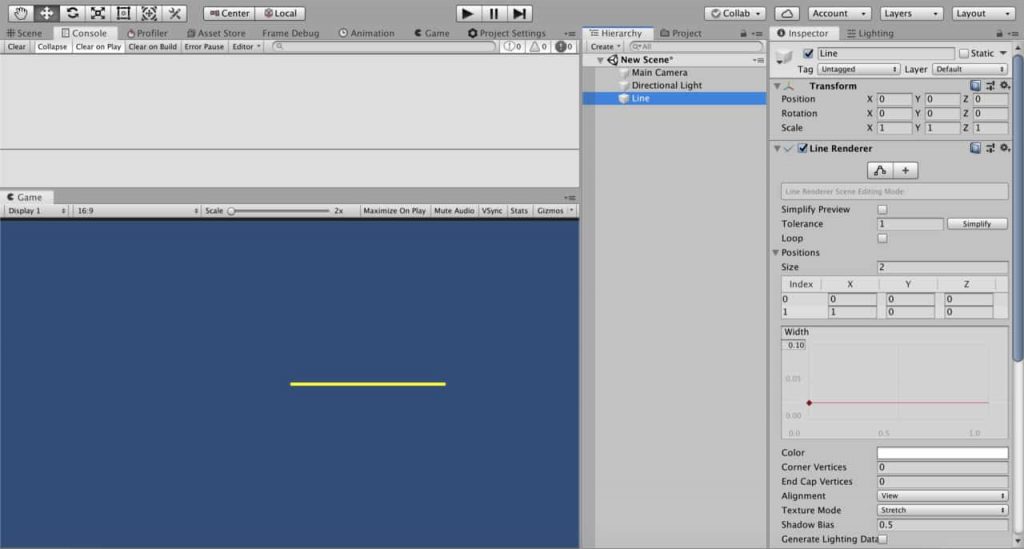
Besides, two draw a triangle, you demand 3 points and to draw a rectangle you demand iv points. Let'southward draw a rectangle as an example.
To draw a rectangle, we need to set positions of 4 points. Nosotros also accept to check the Loop toggle to obtain a closed shape.
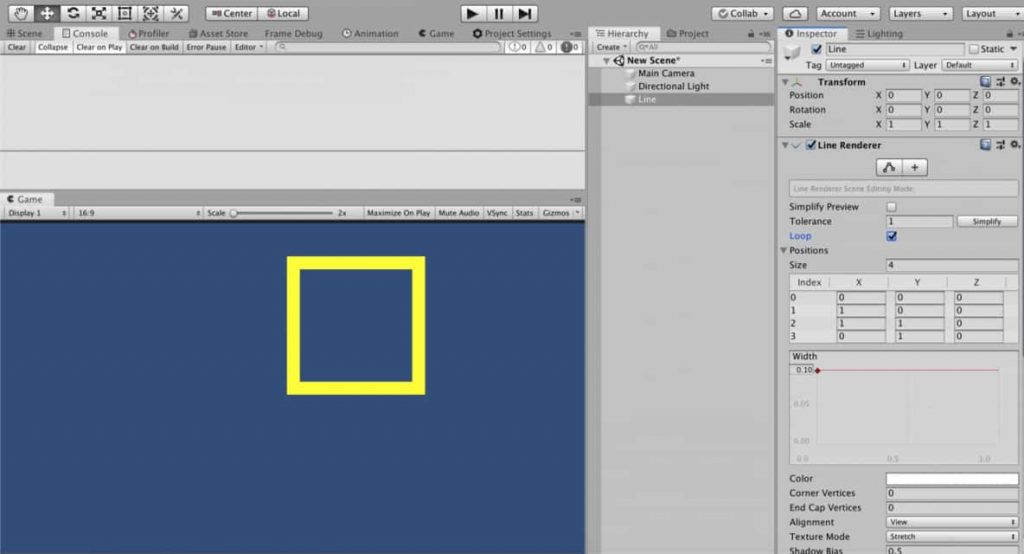
Cartoon Lines From C# Script
If nosotros desire to draw or control lines in real-time, we demand to create a C# script. To draw lines from a script, we determine the size of position array and coordinates of positions in C# script. Therefore, LineRenderer tin can connect the points.
Let'southward draw a triangle using a script as an case. Offset, create a script with the name "DrawScript". And attach this script to a game object which already has a LineRenderer component.
public form DrawScript : MonoBehaviour { private LineRenderer lineRenderer; void Beginning( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ 3 ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - 1 , ane , 0 ) , new Vector3( 1 , 1 , 0 ) } ; DrawTriangle(positions) ; } void DrawTriangle(Vector3[ ] vertexPositions) { lineRenderer.positionCount = 3 ; lineRenderer.SetPositions(vertexPositions) ; } }
This script will draw a triangle. Note that we already set the line width to 0.1 and checked the loop toggle, before. Therefore the same setting is too valid here.
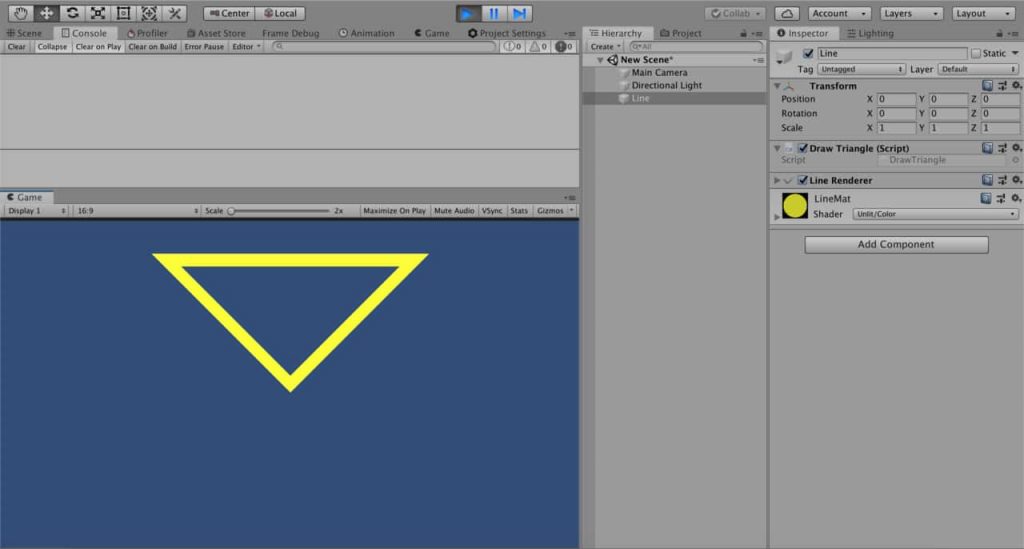
Nosotros tin can also modify the line width from the script using startWidth and endWidth. In addition to this, if you would like to modify line width by position, you tin set different values to them. In this example, Line Renderer volition interpolate the line width according to position.
public class DrawScript : MonoBehaviour { private LineRenderer lineRenderer; void Offset( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ 3 ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - 1 , i , 0 ) , new Vector3( 1 , i , 0 ) } ; DrawTriangle(positions, 0.02 f , 0.02 f ) ; } void DrawTriangle(Vector3[ ] vertexPositions, float startWidth, float endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = true ; lineRenderer.positionCount = 3 ; lineRenderer.SetPositions(vertexPositions) ; } }
Drawing Regular Polygons and Circles
In this section, we are going to come across how we can write a method that draws regular polygons. Since circles are n-gons which has big n, our function will be useful for circles likewise. But starting time, permit me explicate the mathematics backside it.
Vertices of regular polygons are on a circumvolve. Also, the middle of the circle and the center of the polygon are elevation of each other. The most reliable method to describe a polygon is to observe the angle between successive vertices and locate the vertices on the circumvolve. For instance, angle of the arc between successive vertices of a pentagon is 72 degrees or for an octagon, it is 45 degrees. To find this bending, nosotros can carve up 360 degrees(or 2xPI radians) with the number of vertices.
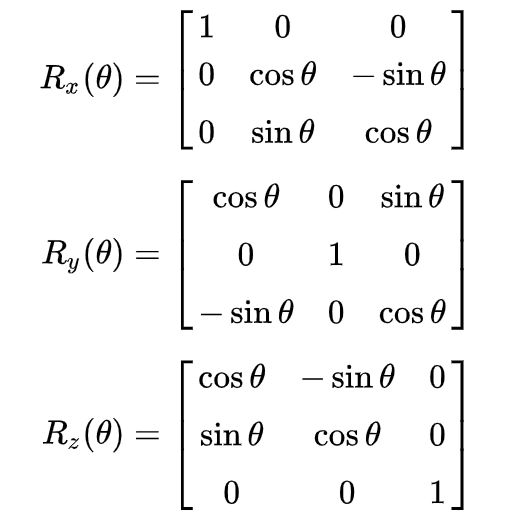
Then we need to find the positions of the vertices. To do this we assign an initial indicate for the commencement vertex and rotate this vertex for each vertex using a rotation matrix.
As y'all probably know, in order to rotate a betoken around an axis, we multiply the position vector of the bespeak with the rotation matrix. Rotation matrices for rotations effectually 10, y and z axes are given on the correct.
For instance, when nosotros want to rotate a point by 90 degrees around the z-axis, which has a coordinate (1,0,0), nosotros multiply the position vector by a rotation matrix.

We demand to construct a rotation matrix to rotate each vertex around the z-axis. Permit'due south me write our DrawPolygon method first and explain it.
void DrawPolygon( int vertexNumber, float radius, Vector3 centerPos, bladder startWidth, float endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = truthful ; float bending = two * Mathf.PI / vertexNumber; lineRenderer.positionCount = vertexNumber; for ( int i = 0 ; i < vertexNumber; i+ + ) { Matrix4x4 rotationMatrix = new Matrix4x4( new Vector4(Mathf.Cos(angle * i) , Mathf.Sin(bending * i) , 0 , 0 ) , new Vector4( - 1 * Mathf.Sin(angle * i) , Mathf.Cos(angle * i) , 0 , 0 ) , new Vector4( 0 , 0 , ane , 0 ) , new Vector4( 0 , 0 , 0 , 1 ) ) ; Vector3 initialRelativePosition = new Vector3( 0 , radius, 0 ) ; lineRenderer.SetPosition(i, centerPos + rotationMatrix.MultiplyPoint(initialRelativePosition) ) ; } }
You may wonder why the constructed rotation matrix is 4×four. In computer graphics, the 3-dimensional earth is represented equally 4-dimensional but this topic is not related to our business hither. We just utilize information technology as if it is 3-dimensional.
Nosotros set the position of initial vertex and rotate it using rotationMatrix each fourth dimension and add the center position to it.
The following image is an example of a hexagon which is drawn by this method.
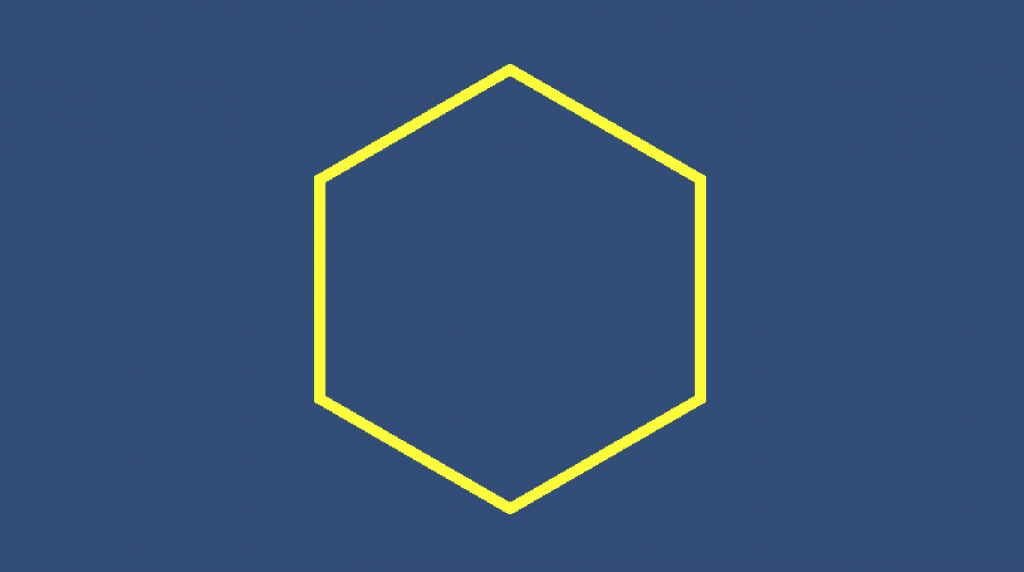
If you increment the number of vertex points, this polygon turns to a circle.
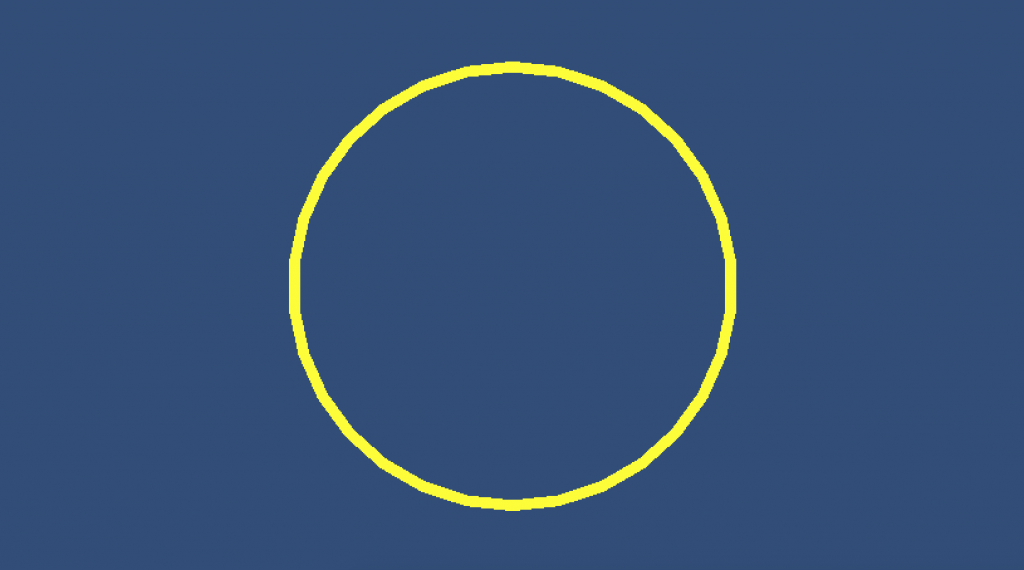
Drawing Waves
In this section, we are going to draw a sinusoidal wave, a traveling wave and a standing wave using sine part.
The mathematical part of the sine wave is given by the following:
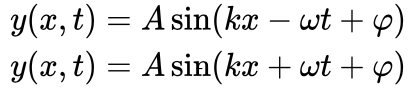
where
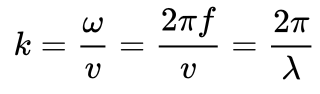
Here, thou is moving ridge number, f is frequency, ω is the angular frequency, λ is wavelength, v is the linear speed, t is the fourth dimension and φ is the phase angle. Nosotros will non worry about the phase angle in our discussions.
Sine wave equation with a minus sign represents traveling moving ridge from left to right and the equation with plus sign represents a traveling line wave correct to left.
In club to draw a stable sinusoidal moving ridge, we can drib the fourth dimension part. The following method will draw a sine moving ridge.
void DrawSineWave(Vector3 startPoint, float amplitude, float wavelength) { float x = 0f; bladder y; float k = 2 * Mathf.PI / wavelength; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * Mathf.Sin(one thousand * 10) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
Our DrawSineWave method takes three parameters. They are startPoint which is for setting the start position in world space, aamplitude which is for setting the amplitude of the wave and wavelength which is for setting the wavelength of the sine wave.
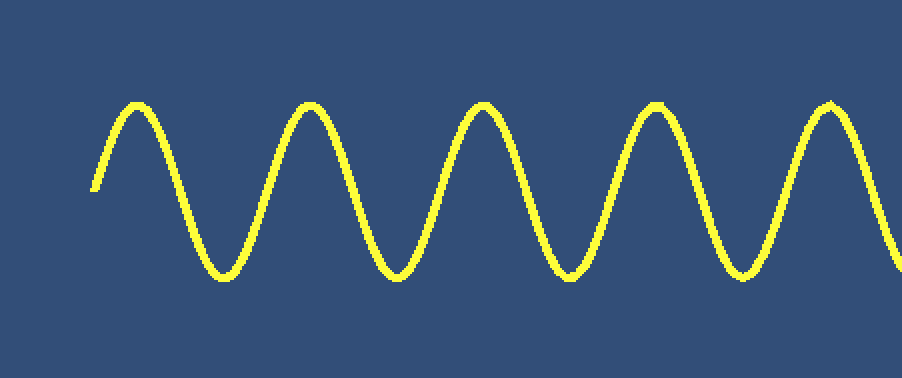
To obtain the positions of the corresponding mathematical office, first, we determine the positions on the x-axis. For each ten, nosotros have to summate the y-position.
To breathing this moving ridge, nosotros have to implement time to our part every bit follows:
void DrawTravellingSineWave(Vector3 startPoint, float amplitude, float wavelength, bladder waveSpeed) { float x = 0f; bladder y; float k = ii * Mathf.PI / wavelength; float westward = k * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * Mathf.Sin(k * x + w * Time.time) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
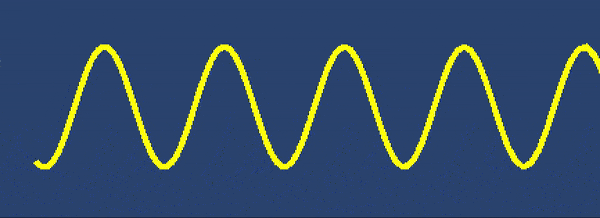
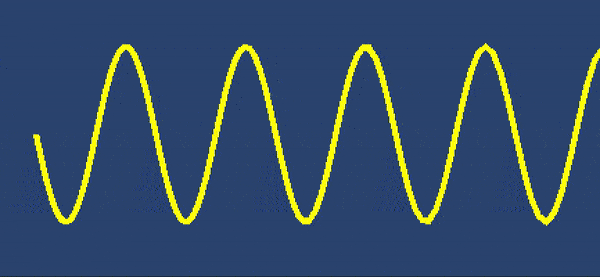
This time we accept four parameters. The quaternary parameter is to gear up the moving ridge speed. This wave travels to the left since we used plus sign in the function. If we would like to create a wave that travels to the right, we have to use the minus sign. You lot should go on in mind that we have to write this method in Update().
To create a standing wave, nosotros have to add two waves which travel to the right and which travel to left.
void DrawStandingSineWave(Vector3 startPoint, float amplitude, float wavelength, bladder waveSpeed) { float x = 0f; float y; float k = 2 * Mathf.PI / wavelength; bladder due west = k * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = aamplitude * (Mathf.Sin(chiliad * 10 + due west * Fourth dimension.time) + Mathf.Sin(g * x - w * Fourth dimension.time) ) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
Cartoon Bézier Curves
Bézier curves are parametric curves that are used to create smoothen curved shapes. They are widely used in reckoner graphics. In this section, we are going to see how nosotros can draw Bézier curves.
When a Bézier curve is controlled by 3 points, and so it is called Quadratic Bézier Curve(the first equation beneath) and when information technology is controlled by iv points, it is called Cubic Bézier Curve.


The following script volition draw a quadratic Bézier bend using positions p0, p1, and p2. You should create iii game objects and assign these objects to corresponding variables in the script to change the shape of the curve in real-time.
using Organisation.Collections; using System.Collections.Generic; using UnityEngine; public class BezierScript : MonoBehaviour { private LineRenderer lineRenderer; public Transform p0; public Transform p1; public Transform p2; void Commencement( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; } void Update( ) { DrawQuadraticBezierCurve(p0.position, p1.position, p2.position) ; } void DrawQuadraticBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2) { lineRenderer.positionCount = 200 ; float t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( 1 - t) * ( 1 - t) * point0 + 2 * ( 1 - t) * t * point1 + t * t * point2; lineRenderer.SetPosition(i, B) ; t + = ( one / ( float )lineRenderer.positionCount) ; } } }
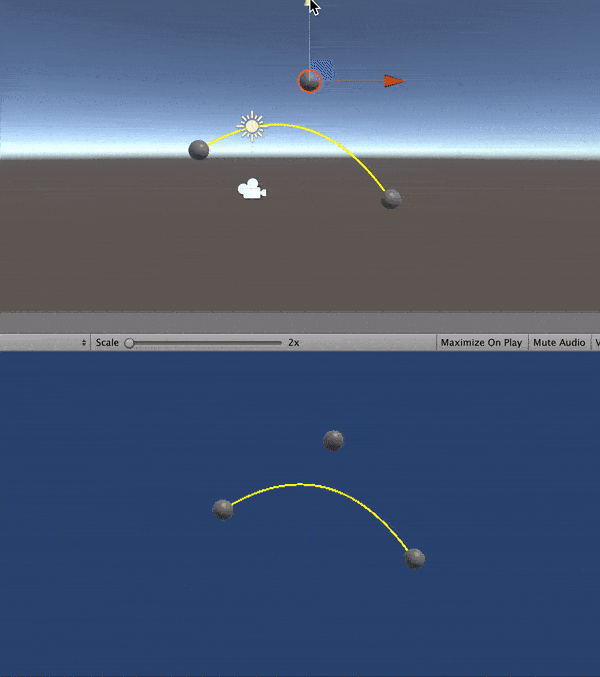
Too, the following method draws a cubic Bézier curve. This fourth dimension we need four points.
void DrawCubicBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2, Vector3 point3) { lineRenderer.positionCount = 200 ; float t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( 1 - t) * ( i - t) * ( 1 - t) * point0 + iii * ( 1 - t) * ( 1 - t) * t * point1 + three * ( ane - t) * t * t * point2 + t * t * t * point3; lineRenderer.SetPosition(i, B) ; t + = ( 1 / ( float )lineRenderer.positionCount) ; } }
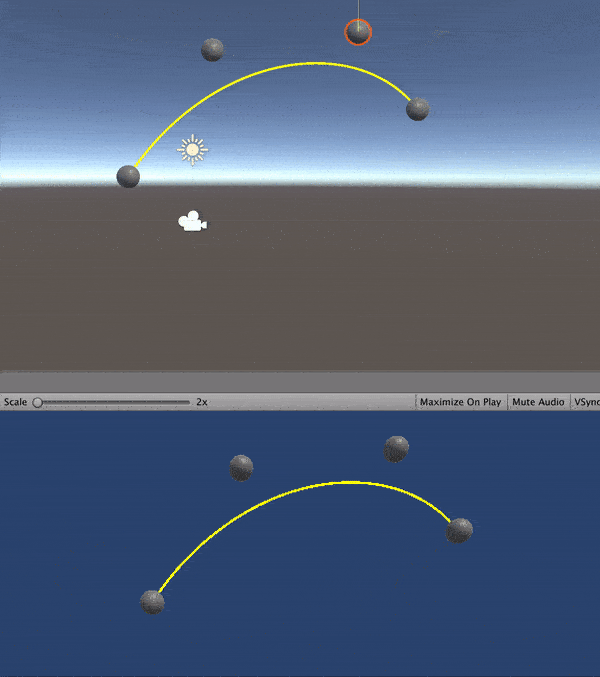
Gratis Drawing using Line Renderer
In this section, we are going to see how we can draw freely using the mouse position. We can do this by creating a new game object with a line renderer attached. When we press the left mouse button, a new game object is created and each frame the position of the mouse added to the line renderer.

Outset of all, we need a prefab to create a new game object when we press the left mouse button. This is an empty game object with a line renderer component attached. In add-on to this, do not forget to assign a material to the line renderer component. Create a prefab from this game object.
Second, create an empty game object and attach the post-obit script DrawManager.
using System.Collections; using System.Collections.Generic; using UnityEngine; public course DrawManager: MonoBehaviour { private LineRenderer lineRenderer; public GameObject drawingPrefab; void Update( ) { if (Input.GetMouseButtonDown( 0 ) ) { GameObject drawing = Instantiate(drawingPrefab) ; lineRenderer = drawing.GetComponent<LineRenderer> ( ) ; } if (Input.GetMouseButton( 0 ) ) { FreeDraw( ) ; } } void FreeDraw( ) { lineRenderer.startWidth = 0.i f ; lineRenderer.endWidth = 0.1 f ; Vector3 mousePos = new Vector3(Input.mousePosition.x, Input.mousePosition.y, 10f) ; lineRenderer.positionCount+ + ; lineRenderer.SetPosition(lineRenderer.positionCount - 1 , Camera.chief.ScreenToWorldPoint(mousePos) ) ; } }
When you printing the left mouse push button, a new game object is instantiated from the prefab which we created before. We get the line renderer component from this game object. Then while we are pressing the left mouse push button, nosotros call FreeDraw() method.
In the FreeDraw method, nosotros have x and y components of the mouse position and fix the z-position as ten. Here, the mouse position is in the screen space coordinates. But we apply world space coordinates in line renderer. Therefore nosotros need to convert mouse position to world space coordinates. In each frame, nosotros as well need to increase the number of points. Since we do not know how many points we demand, we cannot prepare position count before.
References
1-https://docs.unity3d.com/ScriptReference/LineRenderer.html
ii-http://world wide web.theappguruz.com/weblog/bezier-bend-in-games
3-https://en.wikipedia.org/wiki/Bézier_curve
four-https://en.wikipedia.org/wiki/Sine_wave
Source: https://www.codinblack.com/how-to-draw-lines-circles-or-anything-else-using-linerenderer/
0 Response to "draw parabola unity 3d c"
Post a Comment